개요
AJAX(Asynchronous JavaScript and XML)
서버와 통신하기 위해 XMLHttpRequest 객체 사용
비동기성으로 페이지 새로고침을 하지않고도 수행된다.
콜백함수로 구현하며, js에 내장되어 있다.
fetch API
XMLHttpRequest와 유사하나 더 발전된 API (es6 도입)
객체를 Promise 형태로 반환 받는다, json의 타입별로 쉽게 적용이 가능하다.
js에 내장되어 있으며 결과를 json 파싱해서 전달은 한번 더 해줘야 한다.
axios
가장 널리 쓰이는 http 통신 라이브러리
Vue/React 에서도 권고 라이브러리로 axios가 지정이 되어 있다.
Promise 형태 리턴, 외부 라이브러리를 사용하며, 결과가 json으로 파싱되어서 사용하기 편리하다.
외부 라이브러리를 사용하기 때문에 cdn값을 가져와 넣어주어야 한다.
사이트 링크 : https://axios-http.com/kr/docs/intro
시작하기 | Axios Docs
시작하기 브라우저와 node.js에서 사용할 수 있는 Promise 기반 HTTP 클라이언트 라이브러리 Axios란? Axios는 node.js와 브라우저를 위한 Promise 기반 HTTP 클라이언트 입니다. 그것은 동형 입니다(동일한 코
axios-http.com
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
</head>
예제
1. fetch 사용 예제
사이트 내 json을 fetch 방식으로 가져와 파싱하기
코드
const url = "https://jsonplaceholder.typicode.com/todos/";
fetch(url) // url 참조하기
.then((res) => res.json()) // json 형태를 배열로 파싱
.then((res) => console.log(res))
.catch((err) => console.log(err));
출력
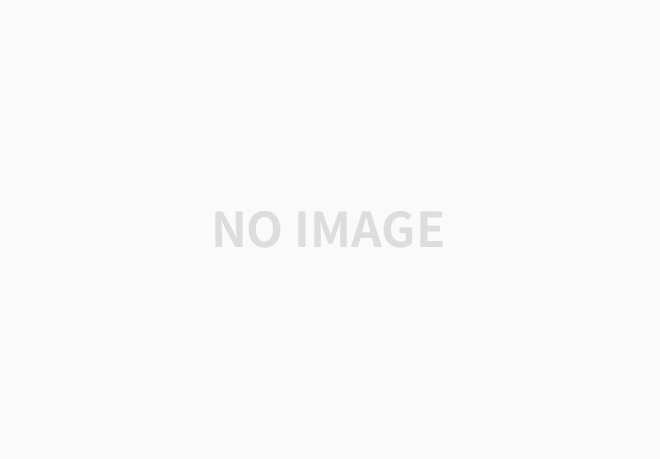
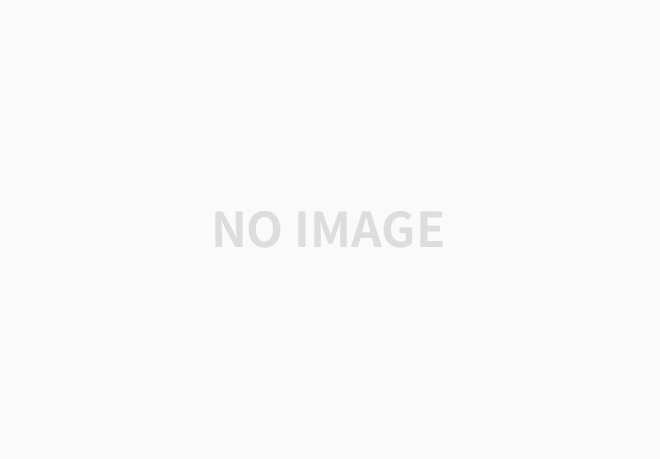
2. axios사용 예제
사이트 내 json을 axios 방식으로 가져와 파싱하기
코드
// 라이브러리를 가져온 후 사용해 줘야 한다.
const url = "https://jsonplaceholder.typicode.com/todos/";
axios.get(url).then((res) => {
console.log(res);
})
출력
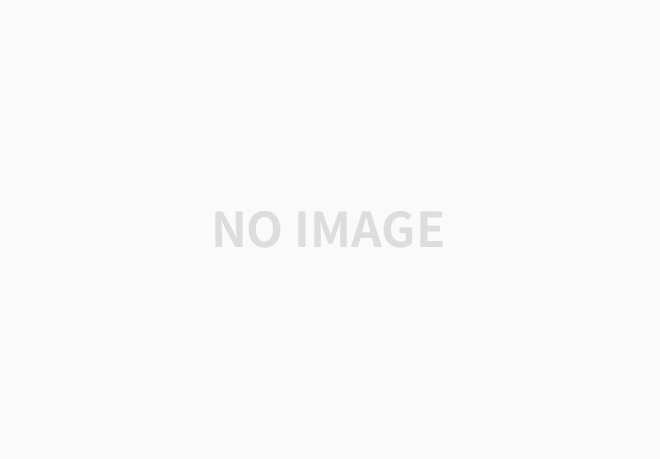
실습
1. axios 활용하기
사이트 내 json을 axios 방식으로 가져와 파싱하고, 특정 값을 변수로 저장하여 사용하기
- https://jsonplaceholder.typicode.com/users/3 호출
- users/3 의 id값을 받아온다
- 받아온 id값을 https://jsonplaceholder.typicode.com/posts?userId=id 에 요청
코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
</head>
<body>
<script>
const url = "https://jsonplaceholder.typicode.com/users/3";
axios.get(url).then((res) => {
let id = res.data.id;
const postUrl = `https://jsonplaceholder.typicode.com/posts?userId=${id}`;
axios.get(postUrl).then(result => {
console.log(result);
})
})
</script>
</body>
</html>
출력
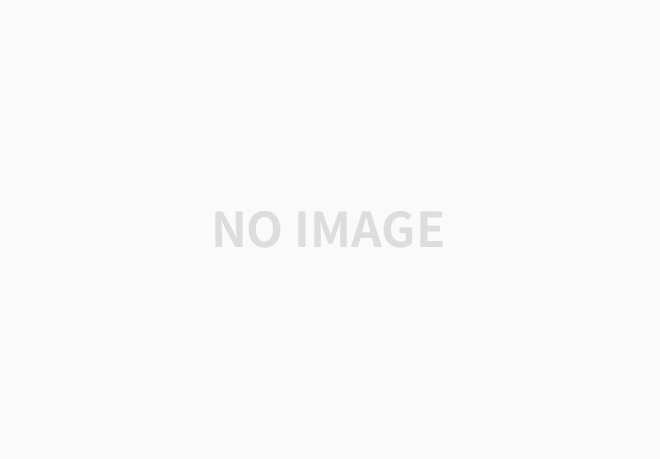
userId가 3인 유저들의 정보를 얻어 올 수 있다.
'웹(WEB) > 자바스크립트(JS)' 카테고리의 다른 글
Node.js 기초 및 설치 (0) | 2024.08.27 |
---|---|
자바스크립트 Axios API 호출 async/await 비동기 작업 웹사이트 구현 (0) | 2024.08.07 |
자바스크립트 Promise (0) | 2024.08.07 |
자바스크립트 Callback 콜백 (0) | 2024.08.06 |
자바스크립트 비동기 프로그래밍 (0) | 2024.08.06 |